Design patterns are an essential part of software development. They provide a way to structure your code, making it more organized, efficient, and easier to maintain. In this blog post, we’ll be taking a look at design patterns in JavaScript and how to use them in your code. We’ll start by discussing the different types of design patterns and their benefits, then dive into some of the more popular ones and show how to use them in your code. By the end of this post, you’ll have a better understanding of design patterns in JavaScript and how to use them to make your code better.
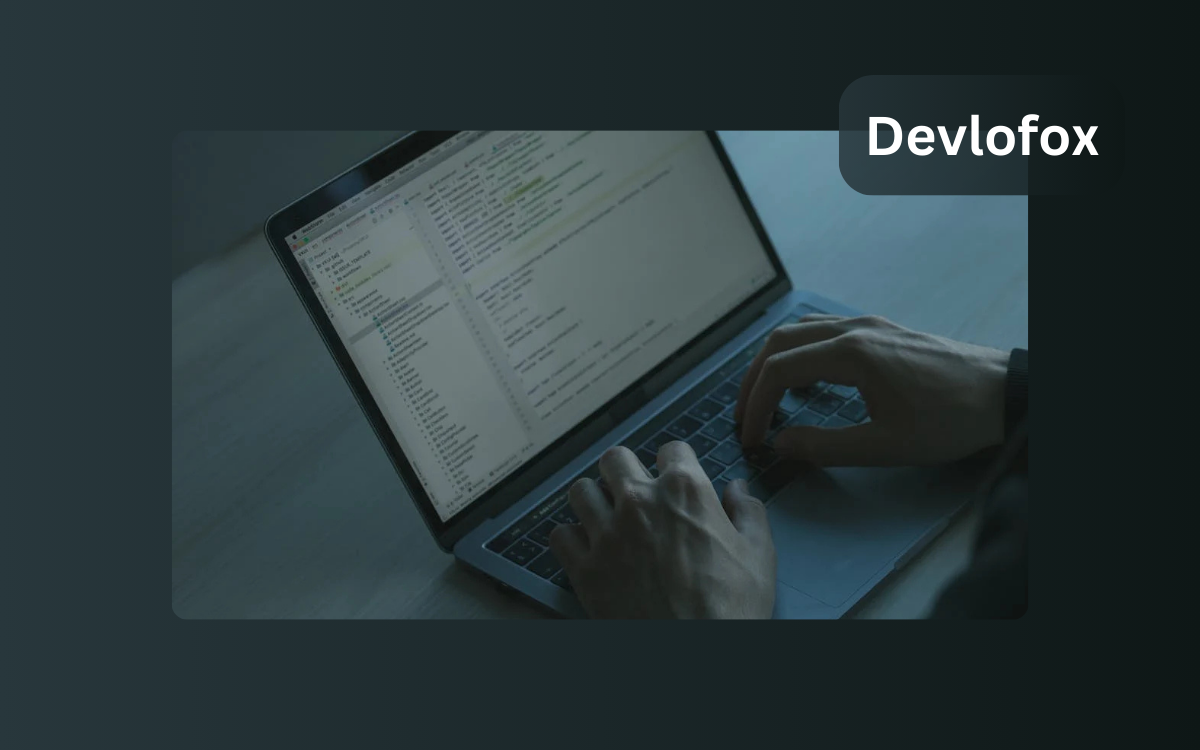
What is a design pattern?
Design patterns are reusable solutions to common programming problems. They are general strategies for how to approach and solve software development challenges. Design patterns provide developers with a structure and guide for how to write efficient, readable, and maintainable code.
Design patterns are more than just lines of code; they are also conceptual approaches to solving problems. By using design patterns, developers can write code that is easier to read, maintain, and extend. Design patterns can be applied to any programming language, from JavaScript to Python to C
Design patterns are often divided into three main categories: creational, structural, and behavioral. Creational patterns focus on the process of creating objects. Structural patterns provide an efficient way to organize data and code. Behavioral patterns describe how objects interact with each other in order to accomplish tasks.
The most popular design pattern in JavaScript is the Module Pattern. This pattern allows developers to create components of code that are self-contained and can easily be reused throughout an application. It also provides encapsulation, meaning that the internal details of each module can remain private and inaccessible from the outside.
When using design patterns, it’s important to understand the purpose behind each pattern and how it can be used in your code. Once you have a good grasp of the different types of design patterns available, you’ll be able to make better decisions when writing your code.
What are the different types of design patterns?
Design patterns are an effective way to create reusable, efficient code that is also maintainable and easily adaptable. A design pattern is a set of instructions or principles that can be used to solve common software development problems. There are many different types of design patterns, each of which offers its own unique advantages and disadvantages.
The most popular design patterns include the following:
1. Singleton Pattern – This pattern is used to ensure that only one instance of a class is created. It’s helpful for creating a global object or accessing a shared resource.
2. Factory Pattern – This pattern provides a way to create objects without needing to specify the exact class of the object that will be created. It’s useful when you need to return an object based on certain criteria.
3. Adapter Pattern – This pattern allows two incompatible interfaces to work together. It’s often used in situations where two libraries need to be integrated, such as when adding a library to an existing application.
4. Strategy Pattern – This pattern allows you to switch between different algorithms or strategies at runtime. It’s helpful when you need to modify the behavior of an algorithm or process without changing the underlying code.
5. Observer Pattern – This pattern enables objects to subscribe to notifications from other objects so they can respond accordingly. It’s useful when you need to ensure multiple objects receive updates in response to a change in state.
6. Command Pattern – This pattern encapsulates a request as an object, allowing the request to be parameterized and stored for later use. It’s helpful when you need to keep track of user requests and execute them at a later time.
Each of these patterns can be used in different situations, depending on your specific needs. Choosing the right design pattern for a given situation can help you create more efficient and maintainable code.
How can I use design patterns in my code?
Design patterns are a powerful way to write efficient, well-structured code. They can be used in any programming language, including JavaScript. Design patterns provide developers with a common language and structure to follow when creating software.
There are several different types of design patterns that can be used in your code. These patterns include the Factory pattern, Singleton pattern, Observer pattern, and Model-View-Controller (MVC) pattern. Each of these patterns serves a different purpose and is suited to different types of applications.
The Factory pattern is used to create objects from classes or functions without having to manually instantiate them. The Singleton pattern is used when you want only one instance of an object to exist at a time. The Observer pattern is used when you want to observe and update data in real-time. The Model-View-Controller pattern is used for separation of concerns between the user interface, business logic, and data layers of an application.
Using design patterns in your code can help make it more maintainable, readable, and extensible. They also help to ensure that code follows the same standard, making it easier for other developers to understand and modify it. To use design patterns in your code, start by deciding which type of design pattern best suits the project you are working on. Then, determine which classes or functions will be needed and what operations they will perform. Next, use the appropriate design pattern to structure your code accordingly. Finally, test and debug your code to ensure it works correctly
What are some benefits of using design patterns?
Design patterns are an important tool for software developers, offering numerous benefits that can help make their lives easier. Design patterns provide developers with an effective way to solve complex problems and simplify their coding process. By using design patterns, developers can create code that is more organized, readable, and easier to maintain.
Design patterns can also help with code reusability. Rather than having to write complex solutions from scratch every time, developers can draw on existing patterns to quickly solve problems. This can greatly reduce development time, allowing developers to focus on other tasks or projects.
Design patterns can also help promote collaboration within a development team. By standardizing the way code is written, design patterns make it easier for different developers to understand each other’s code and work together on projects. This in turn leads to better communication and more successful product launches.
Ultimately, by using design patterns in your code, you can increase the quality of your product while reducing the amount of time it takes to create and maintain it. Design patterns make coding easier and more efficient while helping to ensure consistency and maintainability.
At Devlofox, we don’t just build websites; we build experiences that captivate, engage, and convert.
📞 Call us at +91 7982436893
📩 Email us at Info@devlofox.com
🌐 Visit us: www.devlofox.com
For more details on javascript visit our https://devlofox.com/blog/category/all/ page
Let’s create something extraordinary together! ✨
Contact Devlofox today for a free consultation on how we can build you a stunning and sustainable website!